Will it render?
React rendering cheatsheet
Example 1: Parent component is rendered
In general, a component is rendered if its parent component is rendered (for whatever reason). If the parent is not rendered, then the component will be rendered only if it triggered a re-render with a state setter or uses a context value that changed (more on context below).
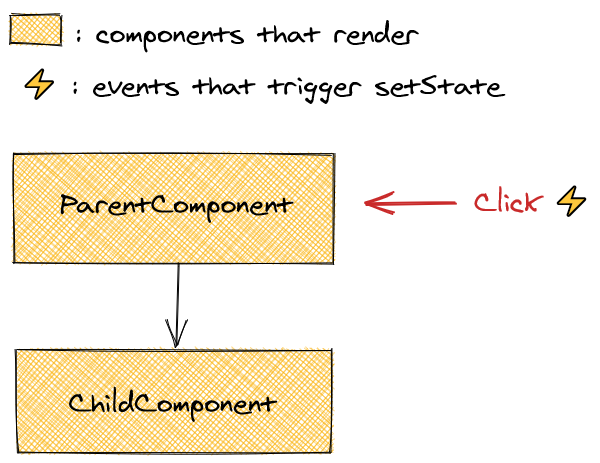
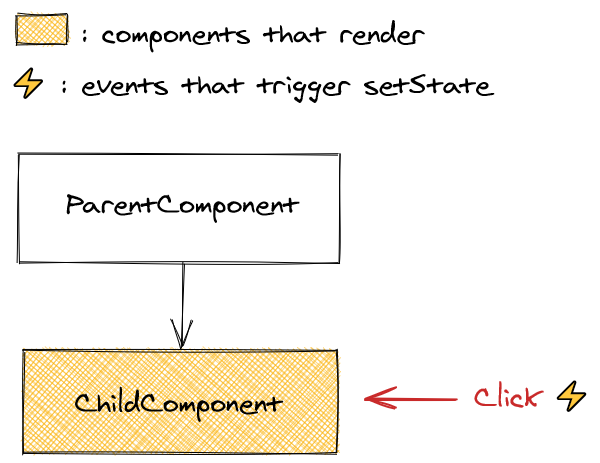
Explore with Codesandbox
Example 2: Context value changed (inefficient)
A component is rendered if it uses a context value and that value changes. However since context naturally comes from a parent, any change triggered by that parent will cause a cascading re-render of the whole app.
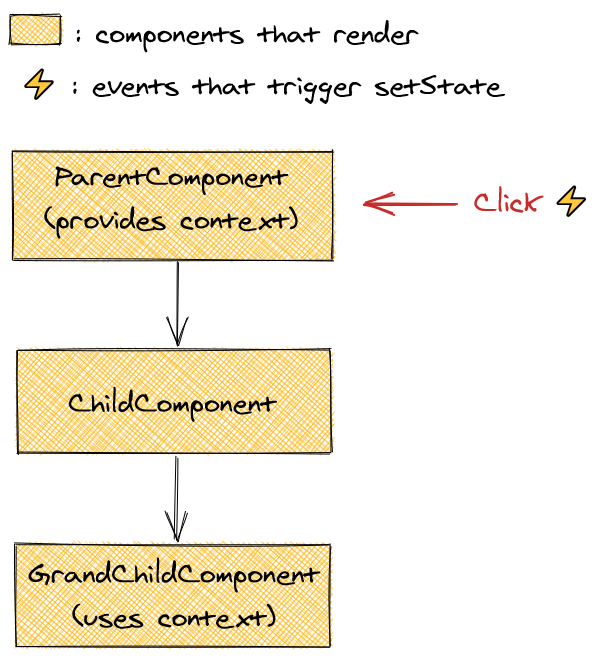
Example 3: Context value changed (efficient)
To avoid cascading re-render we can use the same-element reference technique. Simply speaking, React will not re-render a child if it comes from props and its references the same element across renders. If we put a context provider in another component that takes children props, we can avoid unnecessary renders.
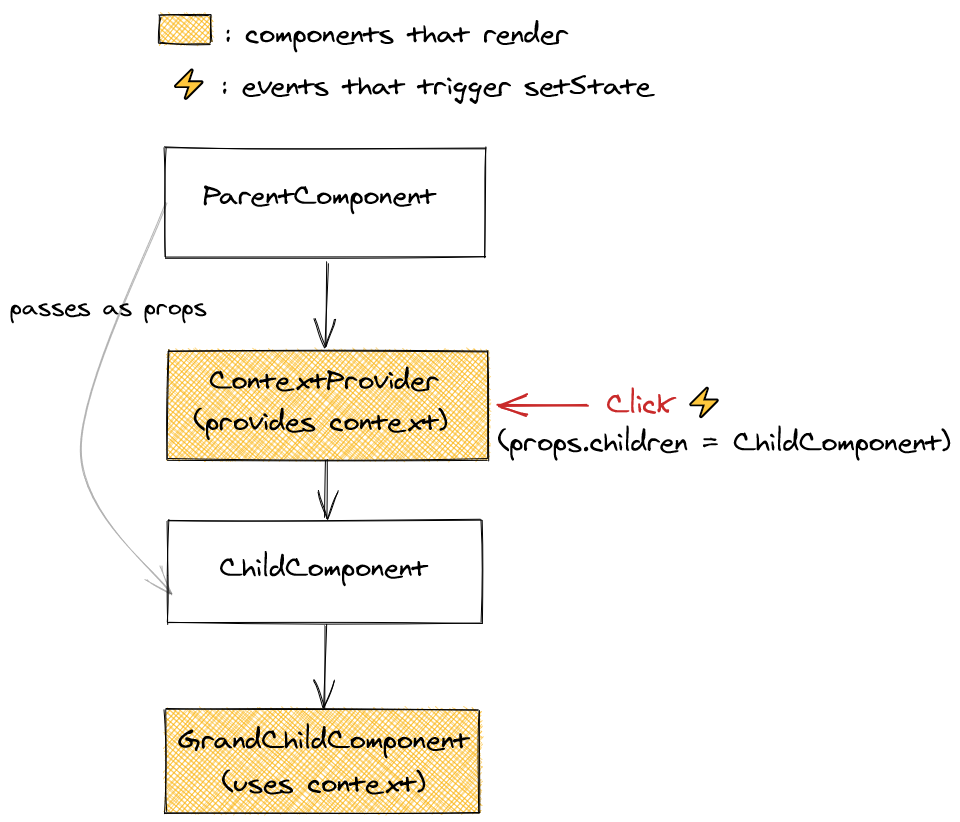
Explore with Codesandbox
Example 4: Meaningless memoization
One way to avoid unnecessary re-render in React is to use React.memo. However it does not make sense if we pass new references to it on each render. Some examples are newly created objects or functions.
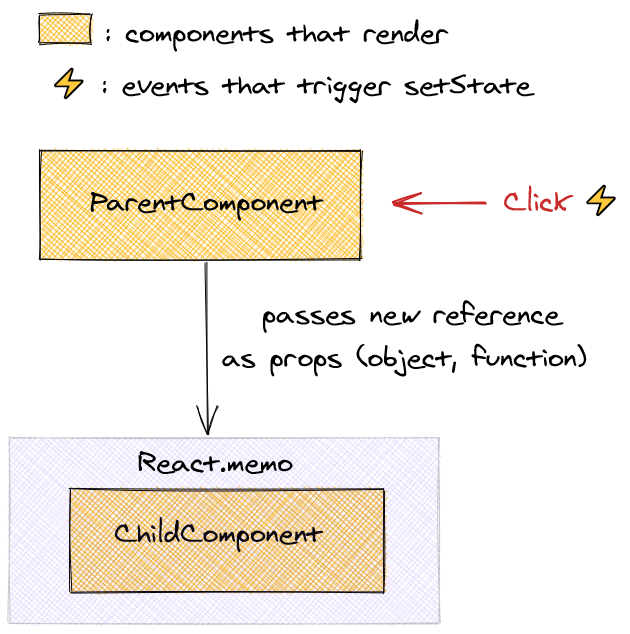
Explore with Codesandbox
Example 5: Meaningful memoization
To make use of React.memo we need to utilize tools that React provides that memoized the very values we pass to React.memo. These are useCallback and useMemo.
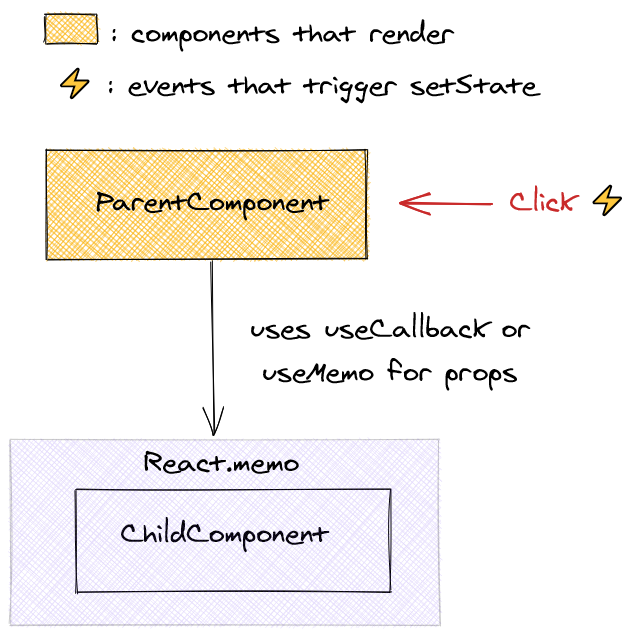
Explore with Codesandbox
Example 6: Context and memoization
Another way to avoid unnecessary re-renders when using Context API is to simply wrap the child of the context provider with React.memo. This way we ensure that only those components which actually need the context value are re-rendered when it changes.
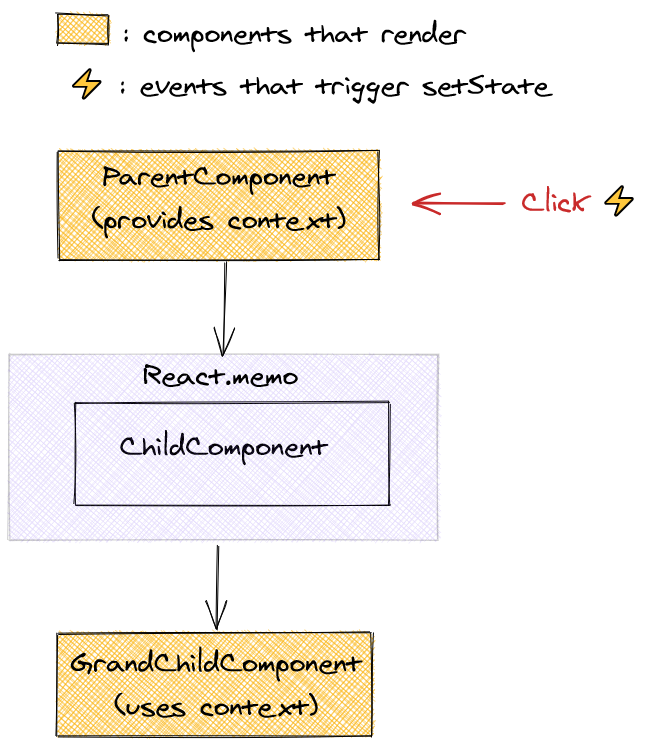